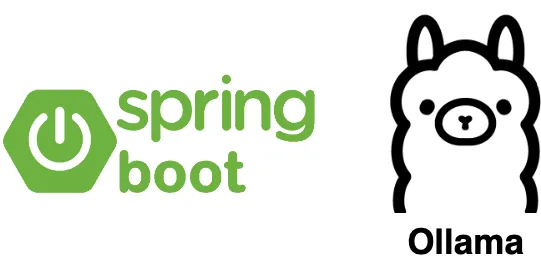
手把手教你用Ollama和Llama3打造Spring Boot AI应用
Ollama在处理自然语言处理(NLP)领域方面非常强大,它能够理解和生成语言,执行与语言相关的多种任务,极大地丰富了人机交互的可能性。ChatClientAppConfig启动Spring Boot应用程序,问以下URL进行测试:也可以在Ollama命令行界面中进行测试。。
Ollama在处理自然语言处理(NLP)领域方面非常强大,它能够理解和生成语言,执行与语言相关的多种任务,极大地丰富了人机交互的可能性。
1 Ollama设置
- 下载与安装: 从官方网站下载ollama:https://ollama.com/
- 启动Ollama服务: 安装完成后,打开浏览器访问http://localhost:11434/ 以启动服务。
- 下载Llama模型: 运行命令“ollama run llama3”以在系统中下载llama3。
2 Spring Boot项目初始化
- 项目初始化: 通过Spring Initializer进行初始化,选择Web和Ollama AI作为项目依赖。
- 实验性提示: 这里需要特别指出,本项目目前处于实验阶段,仅提供快照版本,可能存在不稳定因素。
3 项目结构与配置
- 配置文件: 创建
application.properties
文件以配置项目属性。
spring.application.name=spring-AI
spring.ai.ollama.base-url=http://localhost:11434/api
spring.ai.ollama.model=llama3
server.port=8686
spring.main.allow-bean-definition-overriding=true
- 响应模型: 创建
LlamaResponse
模型以表示服务响应
package com.ai.spring.AI.repsonse;
import lombok.Data;
import lombok.experimental.Accessors;
@Data
@Accessors(chain = true)
public class LlamaResponse {
private String message;
}
- 服务接口: 创建
LlamaAiService
接口
package com.ai.spring.AI.service;
import com.ai.spring.AI.repsonse.LlamaResponse;
public interface LlamaAiService {
LlamaResponse generateMessage(String prompt);
LlamaResponse generateJoke(String topic);
}
- 服务实现: 实现
LlamaAiServiceImpl
package com.ai.spring.AI.service.impl;
import com.ai.spring.AI.repsonse.LlamaResponse;
import com.ai.spring.AI.service.LlamaAiService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class LlamaAiServiceImpl implements LlamaAiService {
@Autowired
private OllamaChatClient chatClient;
@Override
public LlamaResponse generateMessage(String promptMessage) {
final String llamaMessage = chatClient.generate(promptMessage);
return new LlamaResponse().setMessage(llamaMessage);
}
@Override
public LlamaResponse generateJoke(String topic) {
final String llamaMessage = chatClient.generate(String.format("Tell me a joke about %s", topic));
return new LlamaResponse().setMessage(llamaMessage);
}
}
- 客户端接口: 创建
ChatClient
接口
package com.ai.spring.AI.service;
public interface ChatClient {
String generate(String prompt);
}
- Ollama客户端实现: 实现
OllamaChatClient
package com.ai.spring.AI.service.impl;
import com.ai.spring.AI.service.ChatClient;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.web.client.RestTemplate;
import java.util.HashMap;
import java.util.Map;
@Component("customOllamaChatClient")
public class OllamaChatClient implements ChatClient {
private final RestTemplate restTemplate;
private final String baseUrl;
private final String model;
public OllamaChatClient(RestTemplate restTemplate, @Value("${spring.ai.ollama.base-url}") String baseUrl, @Value("${spring.ai.ollama.model}") String model) {
this.restTemplate = restTemplate;
this.baseUrl = baseUrl;
this.model = model;
}
@Override
public String generate(String prompt) {
String url = String.format("%s/generate", baseUrl);
Map<String, Object> request = new HashMap<>();
request.put("model", model);
request.put("prompt", prompt);
request.put("stream", false);
try {
// 打印URL和请求负载以进行调试
System.out.println("URL: " + url);
System.out.println("Request: " + request);
return restTemplate.postForObject(url, request, String.class);
} catch (HttpClientErrorException e) {
// 记录错误详情
System.err.println("Error: " + e.getStatusCode() + " - " + e.getResponseBodyAsString());
throw e;
}
}
}
- 配置类: 创建
AppConfig
以配置RestTemplate
。
package com.ai.spring.AI.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.client.RestTemplate;
@Configuration
public class AppConfig {
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
- 控制器: 创建
LlamaRestController
package com.ai.spring.AI.controller;
import com.ai.spring.AI.repsonse.LlamaResponse;
import com.ai.spring.AI.service.impl.LlamaAiServiceImpl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
@RestController
public class LlamaRestController {
@Autowired
private LlamaAiServiceImpl llamaAiService;
@GetMapping("api/v1/ai/generate")
public ResponseEntity<LlamaResponse> generate(
@RequestParam(value = "promptMessage", defaultValue = "Why is the sky blue?")
String promptMessage) {
final LlamaResponse aiResponse = llamaAiService.generateMessage(promptMessage);
return ResponseEntity.status(HttpStatus.OK).body(aiResponse);
}
@PostMapping("api/v1/ai/generate/joke/{topic}")
public ResponseEntity<LlamaResponse> generateJoke(@PathVariable String topic) {
final LlamaResponse aiResponse = llamaAiService.generateJoke(topic);
return ResponseEntity.status(HttpStatus.OK).body(aiResponse);
}
}
4 启动与测试
启动Spring Boot应用程序,问以下URL进行测试:
-
http://localhost:8686/api/v1/ai/generate/joke/student
-
http://localhost:8686/api/v1/ai/generate
也可以在Ollama命令行界面中进行测试。
如何学习AI大模型?
作为一名热心肠的互联网老兵,我决定把宝贵的AI知识分享给大家。 至于能学习到多少就看你的学习毅力和能力了 。我已将重要的AI大模型资料包括AI大模型入门学习思维导图、精品AI大模型学习书籍手册、视频教程、实战学习等录播视频免费分享出来。
这份完整版的大模型 AI 学习资料已经上传CSDN,朋友们如果需要可以微信扫描下方CSDN官方认证二维码免费领取【保证100%免费
】
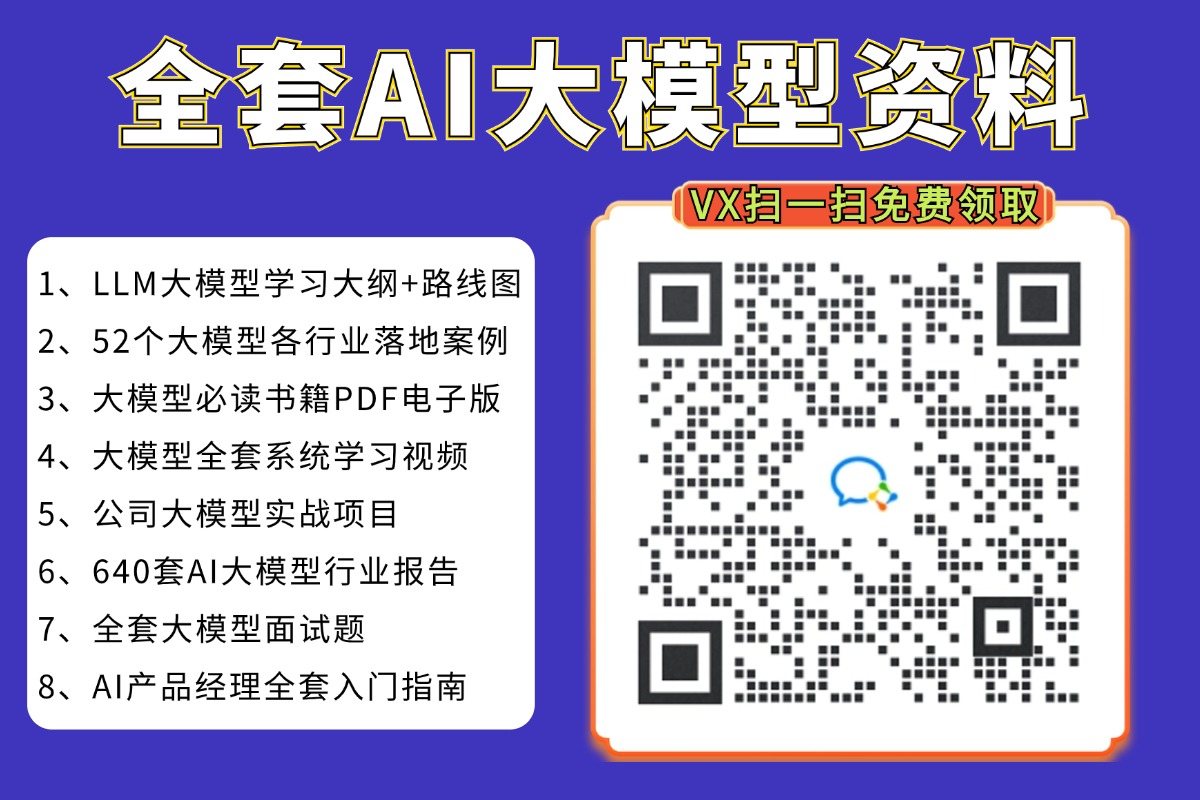
一、全套AGI大模型学习路线
AI大模型时代的学习之旅:从基础到前沿,掌握人工智能的核心技能!
二、640套AI大模型报告合集
这套包含640份报告的合集,涵盖了AI大模型的理论研究、技术实现、行业应用等多个方面。无论您是科研人员、工程师,还是对AI大模型感兴趣的爱好者,这套报告合集都将为您提供宝贵的信息和启示。
三、AI大模型经典PDF籍
随着人工智能技术的飞速发展,AI大模型已经成为了当今科技领域的一大热点。这些大型预训练模型,如GPT-3、BERT、XLNet等,以其强大的语言理解和生成能力,正在改变我们对人工智能的认识。 那以下这些PDF籍就是非常不错的学习资源。
四、AI大模型商业化落地方案
作为普通人,入局大模型时代需要持续学习和实践,不断提高自己的技能和认知水平,同时也需要有责任感和伦理意识,为人工智能的健康发展贡献力量。
更多推荐
所有评论(0)